Amazon EventBridge
The Amazon EventBridge notification service in Buildkite lets you stream events in real-time from your Buildkite account to your AWS account.
Events
Once you've configured an Amazon EventBridge notification service in Buildkite, the following events are published to the partner event bus:
Detail Type | Description |
---|---|
Build Created | A build has been created |
Build Started | A build has started |
Build Finished | A build has finished |
Job Scheduled | A job has been scheduled |
Job Started | A command step job has started running on an agent |
Job Finished | A job has finished. To check a job's result, use the passed field. The value is true when the job passed, and false otherwise. |
Job Activated | A block step job has been unblocked using the web or API |
Agent Connected | An agent has connected to the API |
Agent Lost | An agent has been marked as lost. This happens when Buildkite stops receiving pings from the agent |
Agent Disconnected | An agent has disconnected. This happens when the agent shuts down and disconnects from the API |
Agent Stopping | An agent is stopping. This happens when an agent is instructed to stop from the API. It first transitions to stopping and finishes any current jobs |
Agent Stopped | An agent has stopped. This happens when an agent is instructed to stop from the API. It can be graceful or forceful |
Agent Blocked | An agent has been blocked. This happens when an agent's IP address is no longer included in the agent token's allowed IP addresses |
Cluster Token Registration Blocked | An attempted agent registration is blocked because the request IP address is not included in the agent token's allowed IP addresses |
Audit Event Logged | An audit event has been logged for the organization |
See build states and job states to learn more about the sequence of these events.
Configuring
In your Buildkite Organization's Notification Settings, add an Amazon EventBridge notification service:

Once you've entered your AWS region and AWS Account ID, a Partner Event Source will be created in your AWS account matching the Partner Event Source Name shown on the settings page:
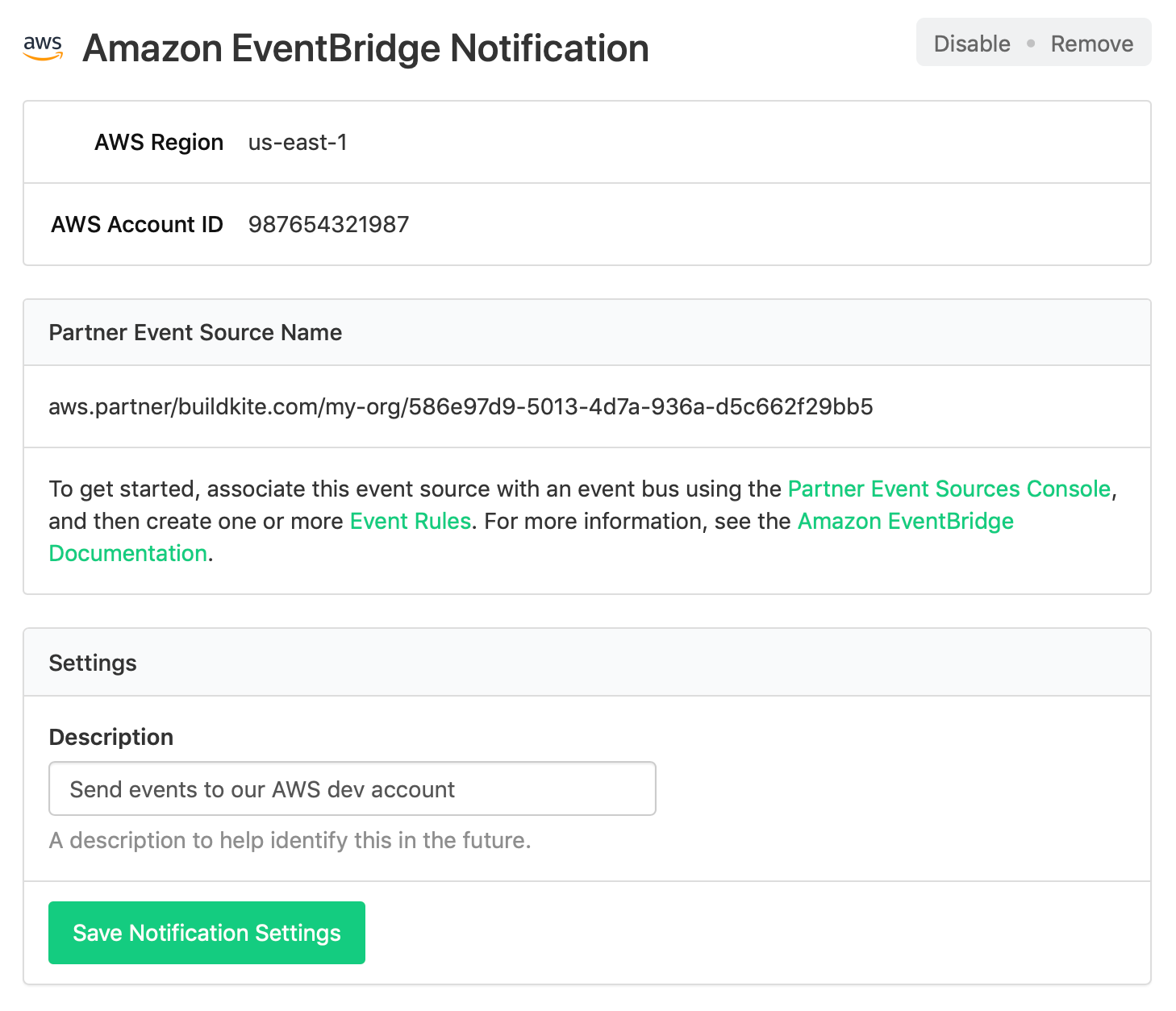
You can then start consuming the events in your AWS account. The links to Partner Event Sources Console and Event Rules take you to the relevant pages in your AWS Console.
Filtering
When creating your EventBridge rule you can specify an Event pattern filter to limit which events will be processed. You can use this to respond only to certain events based on the type, or any attribute from within the event payload.
For example, to only process Build Finished events you'd configure your rule with the following event pattern:
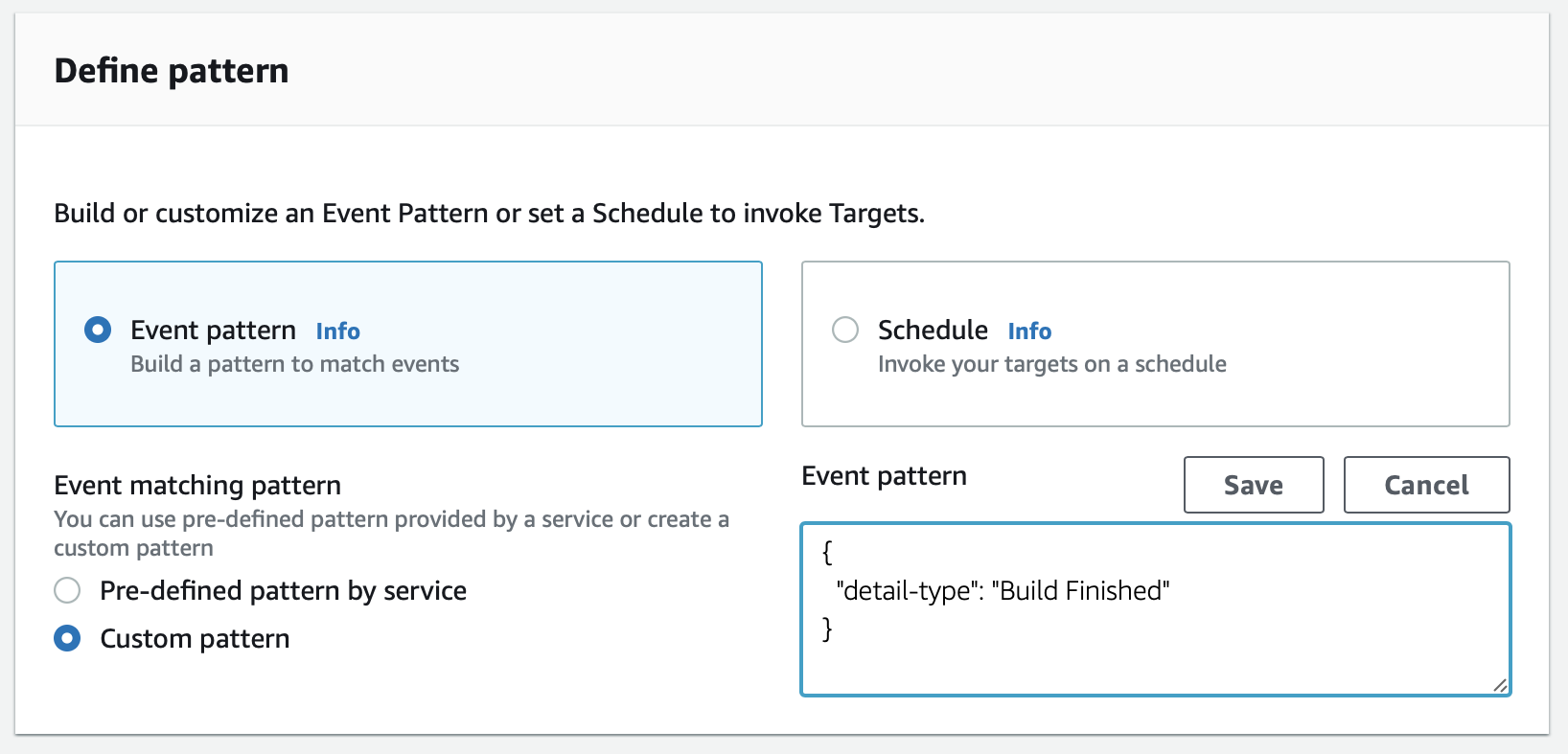
You can use any event property in your custom event pattern. For example, the following event pattern allows only "Build Started" and "Build Finished" events containing a particular pipeline slug:
{
"detail-type": [ "Build Started", "Build Finished" ],
"detail": {
"pipeline": {
"slug": [ "some-pipeline" ]
}
}
}
See the Example Event Payloads for full list of properties, and the AWS EventBridge Event Patterns documentation for full details on the pattern syntax.
Logging
To debug your EventBridge events you can configure a rule to route the event bus directly to AWS CloudWatch Logs:
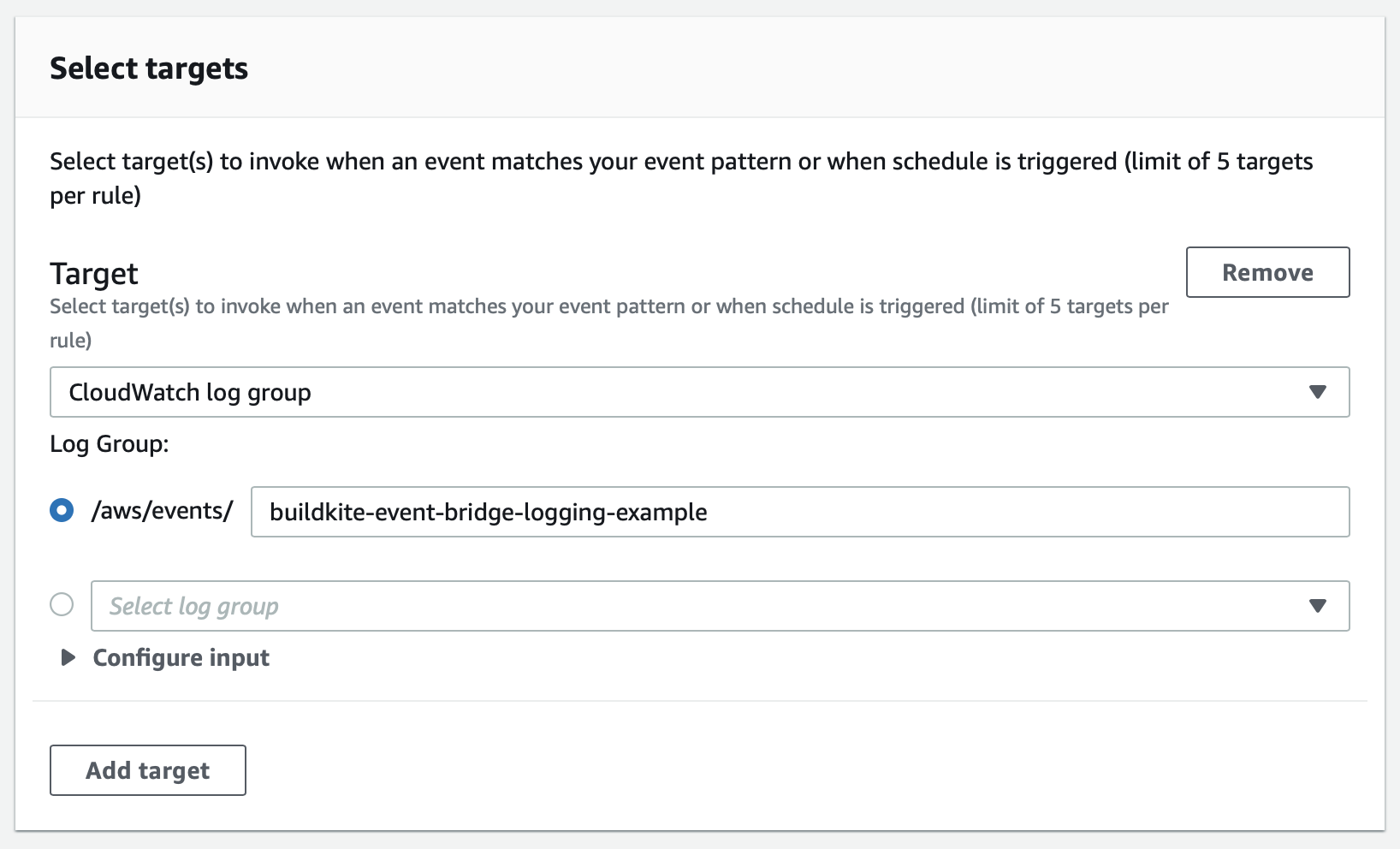
You can then use CloudWatch Logs Insights to query and inspect the live events from your event bus, by choosing the event log group configured above:
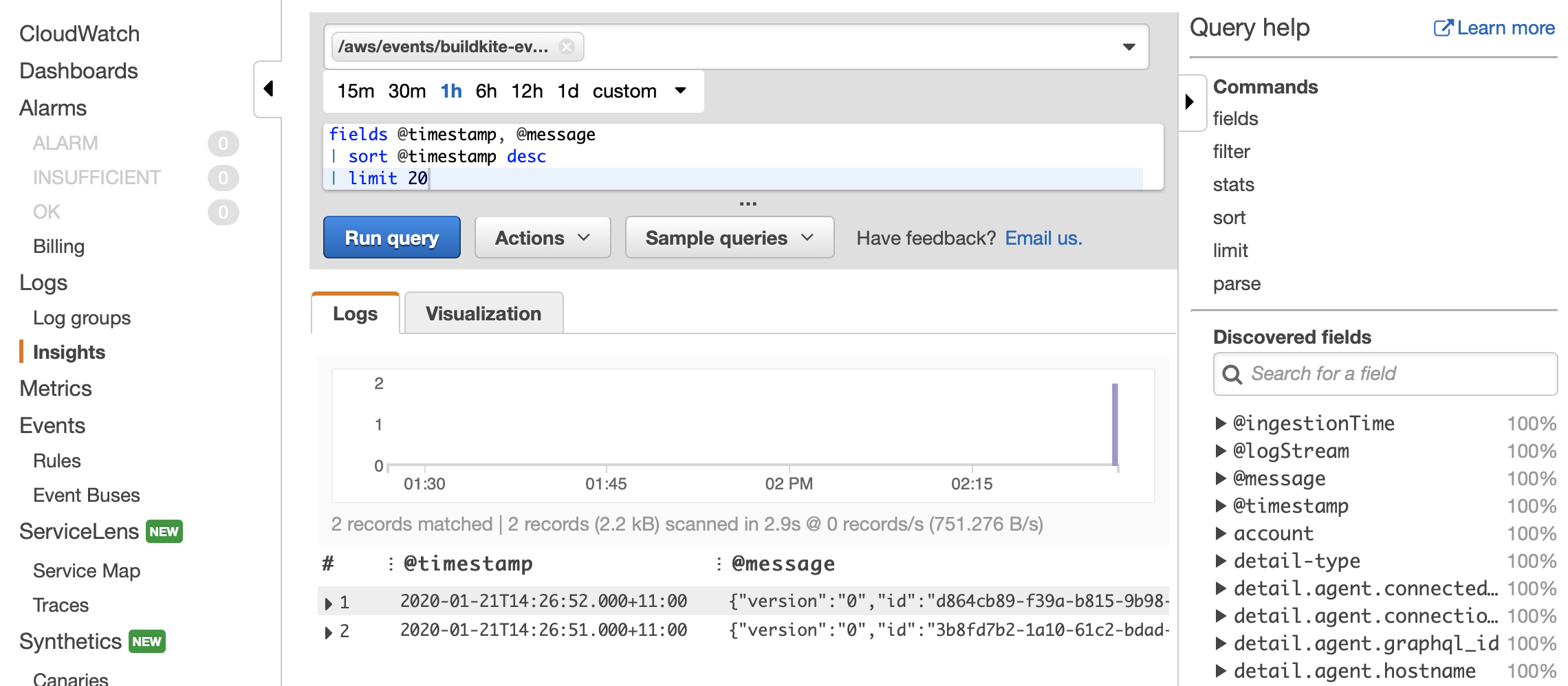
Lambda example: Track agent wait times using CloudWatch metrics
You can use the following AWS Lambda and Job Started event to publish a CloudWatch metric which tracks how long jobs are waiting for agents to become available:
const AWS = require("aws-sdk");
const cloudWatch = new AWS.CloudWatch();
exports.handler = (event, context, callback) => {
const waitTime =
new Date(event.detail.job.started_at) -
new Date(event.detail.job.runnable_at);
console.log(`Job started after waiting ${waitTime} seconds`);
cloudWatch.putMetricData(
{
Namespace: "Buildkite",
MetricData: [
{
MetricName: "Job Agent Wait Time",
Timestamp: new Date(),
StorageResolution: 1,
Unit: "Seconds",
Value: waitTime,
Dimensions: [
{
Name: "Pipeline",
Value: event.detail.pipeline.slug
}
]
}
]
},
(err, data) => {
if (err) console.log(err, err.stack);
callback(null, "Finished");
}
);
};
Official AWS quick start examples
AWS have published three example implementations of using Buildkite with Amazon EventBridge:
Each AWS Quick Start example has a corresponding GitHub repository with full example code.
Example event payloads
AWS EventBridge has strict limits on the size of the payload as documented in Amazon EventBridge quotas. As such, the information included in payloads is restricted to basic information about the event. If you need more information, you can query from the Buildkite APIs using the data in the event.
Build Created
{
"detail-type": "Build Created",
"detail": {
"version": 1,
"build": {
"uuid": "8fcaa7b9-e175-4110-9f48-f79949806a31",
"graphql_id": "QnVpbGQtLS04ZmNhYTdiOS1lMTc1LTQxMTAtOWY0OC1mNzk5NDk4MDZhMzE=",
"number": 123456,
"commit": "5a741616cdf07dc87c5adafe784321eeeb639e33",
"message": "Merge pull request #456 from my-org/chore/update-deps",
"branch": "main",
"state": "scheduled",
"started_at": null,
"finished_at": null,
"source": "webhook"
},
"pipeline": {
"uuid": "88d73553-5533-4f56-9c16-fb38d7817d8f",
"graphql_id": "UGlwZWxpbmUtLS04OGQ3MzU1My01NTMzLTRmNTYtOWMxNi1mYjM4ZDc4MTdkOGY=",
"slug": "my-pipeline",
"repo": "git@somewhere.com:project.git"
},
"organization": {
"uuid": "a98961b7-adc1-41aa-8726-cfb2c46e42e0",
"graphql_id": "T3JnYW5pemF0aW9uLS0tYTk4OTYxYjctYWRjMS00MWFhLTg3MjYtY2ZiMmM0NmU0MmUw",
"slug": "my-org"
}
}
}
{
"detail-type": "Build Started",
"detail": {
"version": 1,
"build": {
"uuid": "8fcaa7b9-e175-4110-9f48-f79949806a31",
"graphql_id": "QnVpbGQtLS04ZmNhYTdiOS1lMTc1LTQxMTAtOWY0OC1mNzk5NDk4MDZhMzE=",
"number": 123456,
"commit": "5a741616cdf07dc87c5adafe784321eeeb639e33",
"message": "Merge pull request #456 from my-org/chore/update-deps",
"branch": "main",
"state": "started",
"started_at": "2019-08-11 06:01:16 UTC",
"finished_at": null,
"source": "webhook"
},
"pipeline": {
"uuid": "88d73553-5533-4f56-9c16-fb38d7817d8f",
"graphql_id": "UGlwZWxpbmUtLS04OGQ3MzU1My01NTMzLTRmNTYtOWMxNi1mYjM4ZDc4MTdkOGY=",
"slug": "my-pipeline",
"repo": "git@somewhere.com:project.git"
},
"organization": {
"uuid": "a98961b7-adc1-41aa-8726-cfb2c46e42e0",
"graphql_id": "T3JnYW5pemF0aW9uLS0tYTk4OTYxYjctYWRjMS00MWFhLTg3MjYtY2ZiMmM0NmU0MmUw",
"slug": "my-org"
}
}
}
Build Finished
{
"detail-type": "Build Finished",
"detail": {
"version": 1,
"build": {
"uuid": "8fcaa7b9-e175-4110-9f48-f79949806a31",
"graphql_id": "QnVpbGQtLS04ZmNhYTdiOS1lMTc1LTQxMTAtOWY0OC1mNzk5NDk4MDZhMzE=",
"number": 123456,
"commit": "5a741616cdf07dc87c5adafe784321eeeb639e33",
"message": "Merge pull request #456 from my-org/chore/update-deps",
"branch": "main",
"state": "passed",
"started_at": "2019-08-11 06:01:16 UTC",
"finished_at": "2019-08-11 06:01:35 UTC",
"source": "webhook"
},
"pipeline": {
"uuid": "88d73553-5533-4f56-9c16-fb38d7817d8f",
"graphql_id": "UGlwZWxpbmUtLS04OGQ3MzU1My01NTMzLTRmNTYtOWMxNi1mYjM4ZDc4MTdkOGY=",
"slug": "my-pipeline",
"repo": "git@somewhere.com:project.git"
},
"organization": {
"uuid": "a98961b7-adc1-41aa-8726-cfb2c46e42e0",
"graphql_id": "T3JnYW5pemF0aW9uLS0tYTk4OTYxYjctYWRjMS00MWFhLTg3MjYtY2ZiMmM0NmU0MmUw",
"slug": "my-org"
}
}
}
Build Blocked
{
"version": "0",
"id": "...",
"detail-type": "Build Finished",
"source": "...",
"account": "...",
"time": "2022-01-30T04:32:06Z",
"region": "us-east-1",
"resources": [],
"detail": {
"version": 1,
"build": {
"uuid": "...",
"graphql_id": "...",
"number": 23,
"commit": "...",
"message": "Update index.html",
"branch": "main",
"state": "blocked",
"blocked_state": null,
"source": "ui",
"started_at": "2022-01-30 04:31:59 UTC",
"finished_at": "2022-01-30 04:32:06 UTC"
},
"pipeline": {
"uuid": "...",
"graphql_id": "...",
"slug": "webhook-test",
"repo": "git@github.com:nithyaasworld/add-contact-chip.git"
},
"organization": {
"uuid": "...",
"graphql_id": "...",
"slug": "nithya-bk"
}
}
}
Job Scheduled
{
"detail-type": "Job Scheduled",
"detail": {
"version": 1,
"job": {
"uuid": "9e6c3f19-4fdb-4e8e-b925-28cd7504e17f",
"graphql_id": "Sm9iLS0tOWU2YzNmMTktNGZkYi00ZThlLWI5MjUtMjhjZDc1MDRlMTdm",
"type": "script",
"label": ":nodejs: Test",
"step_key": "node_test",
"command": "yarn test",
"agent_query_rules": [
"queue=default"
],
"exit_status": null,
"passed": false,
"soft_failed": false,
"state": "assigned",
"runnable_at": "2019-08-11 06:01:14 UTC",
"started_at": null,
"finished_at": null,
"unblocked_by": null
},
"build": {
"uuid": "8fcaa7b9-e175-4110-9f48-f79949806a31",
"graphql_id": "QnVpbGQtLS04ZmNhYTdiOS1lMTc1LTQxMTAtOWY0OC1mNzk5NDk4MDZhMzE=",
"number": 123456,
"commit": "5a741616cdf07dc87c5adafe784321eeeb639e33",
"message": "Merge pull request #456 from my-org/chore/update-deps",
"branch": "main",
"state": "started",
"source": "webhook"
},
"pipeline": {
"uuid": "88d73553-5533-4f56-9c16-fb38d7817d8f",
"graphql_id": "UGlwZWxpbmUtLS04OGQ3MzU1My01NTMzLTRmNTYtOWMxNi1mYjM4ZDc4MTdkOGY=",
"slug": "my-pipeline",
"repo": "git@somewhere.com:project.git"
},
"organization": {
"uuid": "a98961b7-adc1-41aa-8726-cfb2c46e42e0",
"graphql_id": "T3JnYW5pemF0aW9uLS0tYTk4OTYxYjctYWRjMS00MWFhLTg3MjYtY2ZiMmM0NmU0MmUw",
"slug": "my-org"
}
}
}
Job Started
{
"detail-type": "Job Started",
"detail": {
"version": 1,
"job": {
"uuid": "9e6c3f19-4fdb-4e8e-b925-28cd7504e17f",
"graphql_id": "Sm9iLS0tOWU2YzNmMTktNGZkYi00ZThlLWI5MjUtMjhjZDc1MDRlMTdm",
"type": "script",
"label": ":nodejs: Test",
"step_key": "node_test",
"command": "yarn test",
"agent_query_rules": [
"queue=default"
],
"exit_status": null,
"passed": false,
"soft_failed": false,
"state": "started",
"runnable_at": "2019-08-11 06:01:14 UTC",
"started_at": "2019-08-11 06:01:16 UTC",
"finished_at": null,
"unblocked_by": null
},
"build": {
"uuid": "8fcaa7b9-e175-4110-9f48-f79949806a31",
"graphql_id": "QnVpbGQtLS04ZmNhYTdiOS1lMTc1LTQxMTAtOWY0OC1mNzk5NDk4MDZhMzE=",
"number": 123456,
"commit": "5a741616cdf07dc87c5adafe784321eeeb639e33",
"message": "Merge pull request #456 from my-org/chore/update-deps",
"branch": "main",
"state": "started",
"source": "webhook"
},
"pipeline": {
"uuid": "88d73553-5533-4f56-9c16-fb38d7817d8f",
"graphql_id": "UGlwZWxpbmUtLS04OGQ3MzU1My01NTMzLTRmNTYtOWMxNi1mYjM4ZDc4MTdkOGY=",
"slug": "my-pipeline",
"repo": "git@somewhere.com:project.git"
},
"organization": {
"uuid": "a98961b7-adc1-41aa-8726-cfb2c46e42e0",
"graphql_id": "T3JnYW5pemF0aW9uLS0tYTk4OTYxYjctYWRjMS00MWFhLTg3MjYtY2ZiMmM0NmU0MmUw",
"slug": "my-org"
}
}
}
Job Finished
These types of events may contain a signal_reason
field value.
{
"detail-type": "Job Finished",
"detail": {
"version": 1,
"job": {
"uuid": "9e6c3f19-4fdb-4e8e-b925-28cd7504e17f",
"graphql_id": "Sm9iLS0tOWU2YzNmMTktNGZkYi00ZThlLWI5MjUtMjhjZDc1MDRlMTdm",
"type": "script",
"label": ":nodejs: Test",
"step_key": "node_test",
"command": "yarn test",
"agent_query_rules": [
"queue=default"
],
"exit_status": 0,
"signal_reason": "see-reason-below",
"passed": true,
"soft_failed": false,
"state": "finished",
"runnable_at": "2019-08-11 06:01:14 UTC",
"started_at": "2019-08-11 06:01:16 UTC",
"finished_at": "2019-08-11 06:01:35 UTC",
"unblocked_by": null
},
"build": {
"uuid": "8fcaa7b9-e175-4110-9f48-f79949806a31",
"graphql_id": "QnVpbGQtLS04ZmNhYTdiOS1lMTc1LTQxMTAtOWY0OC1mNzk5NDk4MDZhMzE=",
"number": 123456,
"commit": "5a741616cdf07dc87c5adafe784321eeeb639e33",
"message": "Merge pull request #456 from my-org/chore/update-deps",
"branch": "main",
"state": "started",
"source": "webhook"
},
"pipeline": {
"uuid": "88d73553-5533-4f56-9c16-fb38d7817d8f",
"graphql_id": "UGlwZWxpbmUtLS04OGQ3MzU1My01NTMzLTRmNTYtOWMxNi1mYjM4ZDc4MTdkOGY=",
"slug": "my-pipeline",
"repo": "git@somewhere.com:project.git"
},
"organization": {
"uuid": "a98961b7-adc1-41aa-8726-cfb2c46e42e0",
"graphql_id": "T3JnYW5pemF0aW9uLS0tYTk4OTYxYjctYWRjMS00MWFhLTg3MjYtY2ZiMmM0NmU0MmUw",
"slug": "my-org"
}
}
}
Signal reason in job finished events
The signal_reason
field of a job finished event is only be present when the exit_status
field value in the same event is not 0
. The signal_reason
field's value indicates the reason why a job was either stopped, or why the job never ran.
Signal Reason | Description |
---|---|
agent_refused |
The agent refused to run the job, as it was not allowed by a pre-bootstrap hook |
agent_stop |
The agent was stopped while the job was running |
cancel |
The job was cancelled by a user |
signature_rejected |
The job was rejected due to a mismatch with the step's signature |
process_run_error |
The job failed to start due to an error in the process run. This is usually a bug in the agent, contact support if this is happening regularly. |
Job Activated
{
"detail-type": "Job Activated",
"detail": {
"version": 1,
"job": {
"uuid": "9e6c3f19-4fdb-4e8e-b925-28cd7504e17f",
"graphql_id": "Sm9iLS0tOWU2YzNmMTktNGZkYi00ZThlLWI5MjUtMjhjZDc1MDRlMTdm",
"type": "manual",
"label": ":rocket: Deploy",
"step_key": "manual_deploy",
"command": null,
"agent_query_rules": [],
"exit_status": null,
"passed": false,
"soft_failed": false,
"state": "finished",
"runnable_at": null,
"started_at": null,
"finished_at": null,
"unblocked_by": {
"uuid": "c07c69c6-11d2-4375-9148-9d0338b0a836",
"graphql_id": "VXNlci0tLWMwN2M2OWM2LTExZDItNDM3NS05MTQ4LTlkMDMzOGIwYTgzNg==",
"name": "bell"
}
},
"build": {
"uuid": "8fcaa7b9-e175-4110-9f48-f79949806a31",
"graphql_id": "QnVpbGQtLS04ZmNhYTdiOS1lMTc1LTQxMTAtOWY0OC1mNzk5NDk4MDZhMzE=",
"number": 123456,
"commit": "5a741616cdf07dc87c5adafe784321eeeb639e33",
"message": "Merge pull request #456 from my-org/chore/update-deps",
"branch": "main",
"state": "started",
"source": "webhook"
},
"pipeline": {
"uuid": "88d73553-5533-4f56-9c16-fb38d7817d8f",
"graphql_id": "UGlwZWxpbmUtLS04OGQ3MzU1My01NTMzLTRmNTYtOWMxNi1mYjM4ZDc4MTdkOGY=",
"slug": "my-pipeline",
"repo": "git@somewhere.com:project.git"
},
"organization": {
"uuid": "a98961b7-adc1-41aa-8726-cfb2c46e42e0",
"graphql_id": "T3JnYW5pemF0aW9uLS0tYTk4OTYxYjctYWRjMS00MWFhLTg3MjYtY2ZiMmM0NmU0MmUw",
"slug": "my-org"
}
}
}
Agent Connected
{
"detail-type": "Agent Connected",
"detail": {
"version": 1,
"agent": {
"uuid": "288139c5-728d-4c22-88e3-5a926b6c4a51",
"graphql_id": "QWdlbnQtLS0yODgxMzljNS03MjhkLTRjMjItODhlMy01YTkyNmI2YzRhNTE=",
"connection_state": "connected",
"name": "my-agent-1",
"version": "3.13.2",
"ip_address": "3.80.193.183",
"hostname": "ip-10-0-2-73.ec2.internal",
"pid": "18534",
"priority": 0,
"meta_data": [
"aws:instance-id=i-0ce2c738afbfc6c83"
],
"connected_at": "2019-08-10 09:44:40 UTC",
"disconnected_at": null,
"lost_at": null
},
"organization": {
"uuid": "a98961b7-adc1-41aa-8726-cfb2c46e42e0",
"graphql_id": "T3JnYW5pemF0aW9uLS0tYTk4OTYxYjctYWRjMS00MWFhLTg3MjYtY2ZiMmM0NmU0MmUw",
"slug": "my-org"
},
"token": {
"uuid": "df75860c-94f9-4275-91cb-3986590f45b5",
"created_at": "2019-08-10 07:44:40 UTC",
"description": "Default agent token"
}
}
}
Agent Disconnected
{
"detail-type": "Agent Disconnected",
"detail": {
"version": 1,
"agent": {
"uuid": "288139c5-728d-4c22-88e3-5a926b6c4a51",
"graphql_id": "QWdlbnQtLS0yODgxMzljNS03MjhkLTRjMjItODhlMy01YTkyNmI2YzRhNTE=",
"connection_state": "disconnected",
"name": "my-agent-1",
"version": "3.13.2",
"ip_address": "3.80.193.183",
"hostname": "ip-10-0-2-73.ec2.internal",
"pid": "18534",
"priority": 0,
"meta_data": [
"aws:instance-id=i-0ce2c738afbfc6c83"
],
"connected_at": "2019-08-10 09:44:40 UTC",
"disconnected_at": "2019-08-10 09:54:40 UTC",
"lost_at": null
},
"organization": {
"uuid": "a98961b7-adc1-41aa-8726-cfb2c46e42e0",
"graphql_id": "T3JnYW5pemF0aW9uLS0tYTk4OTYxYjctYWRjMS00MWFhLTg3MjYtY2ZiMmM0NmU0MmUw",
"slug": "my-org"
},
"token": {
"uuid": "df75860c-94f9-4275-91cb-3986590f45b5",
"created_at": "2019-08-10 07:44:40 UTC",
"description": "Default agent token"
}
}
}
Agent Lost
{
"detail-type": "Agent Lost",
"detail": {
"version": 1,
"agent": {
"uuid": "288139c5-728d-4c22-88e3-5a926b6c4a51",
"graphql_id": "QWdlbnQtLS0yODgxMzljNS03MjhkLTRjMjItODhlMy01YTkyNmI2YzRhNTE=",
"connection_state": "lost",
"name": "my-agent-1",
"version": "3.13.2",
"ip_address": "3.80.193.183",
"hostname": "ip-10-0-2-73.ec2.internal",
"pid": "18534",
"priority": 0,
"meta_data": [
"aws:instance-id=i-0ce2c738afbfc6c83"
],
"connected_at": "2019-08-10 09:44:40 UTC",
"disconnected_at": "2019-08-10 09:54:40 UTC",
"lost_at": "2019-08-10 09:54:40 UTC"
},
"organization": {
"uuid": "a98961b7-adc1-41aa-8726-cfb2c46e42e0",
"graphql_id": "T3JnYW5pemF0aW9uLS0tYTk4OTYxYjctYWRjMS00MWFhLTg3MjYtY2ZiMmM0NmU0MmUw",
"slug": "my-org"
},
"token": {
"uuid": "df75860c-94f9-4275-91cb-3986590f45b5",
"created_at": "2019-08-10 07:44:40 UTC",
"description": "Default agent token"
}
}
}
Agent Stopping
{
"detail-type": "Agent Stopping",
"detail": {
"version": 1,
"agent": {
"uuid": "288139c5-728d-4c22-88e3-5a926b6c4a51",
"graphql_id": "QWdlbnQtLS0yODgxMzljNS03MjhkLTRjMjItODhlMy01YTkyNmI2YzRhNTE=",
"connection_state": "stopping",
"name": "my-agent-1",
"version": "3.13.2",
"ip_address": "3.80.193.183",
"hostname": "ip-10-0-2-73.ec2.internal",
"pid": "18534",
"priority": 0,
"meta_data": [
"aws:instance-id=i-0ce2c738afbfc6c83"
],
"connected_at": "2019-08-10 09:44:40 UTC",
"disconnected_at": null,
"lost_at": null
},
"organization": {
"uuid": "a98961b7-adc1-41aa-8726-cfb2c46e42e0",
"graphql_id": "T3JnYW5pemF0aW9uLS0tYTk4OTYxYjctYWRjMS00MWFhLTg3MjYtY2ZiMmM0NmU0MmUw",
"slug": "my-org"
},
"token": {
"uuid": "df75860c-94f9-4275-91cb-3986590f45b5",
"created_at": "2019-08-10 07:44:40 UTC",
"description": "Default agent token"
}
}
}
Agent Stopped
{
"detail-type": "Agent Stopped",
"detail": {
"version": 1,
"agent": {
"uuid": "288139c5-728d-4c22-88e3-5a926b6c4a51",
"graphql_id": "QWdlbnQtLS0yODgxMzljNS03MjhkLTRjMjItODhlMy01YTkyNmI2YzRhNTE=",
"connection_state": "stopped",
"name": "my-agent-1",
"version": "3.13.2",
"ip_address": "3.80.193.183",
"hostname": "ip-10-0-2-73.ec2.internal",
"pid": "18534",
"priority": 0,
"meta_data": [
"aws:instance-id=i-0ce2c738afbfc6c83"
],
"connected_at": "2019-08-10 09:44:40 UTC",
"disconnected_at": "2019-08-10 09:54:40 UTC",
"lost_at": null
},
"organization": {
"uuid": "a98961b7-adc1-41aa-8726-cfb2c46e42e0",
"graphql_id": "T3JnYW5pemF0aW9uLS0tYTk4OTYxYjctYWRjMS00MWFhLTg3MjYtY2ZiMmM0NmU0MmUw",
"slug": "my-org"
},
"token": {
"uuid": "df75860c-94f9-4275-91cb-3986590f45b5",
"created_at": "2019-08-10 07:44:40 UTC",
"description": "Default agent token"
}
}
}
Agent Blocked
{
"detail-type": "Agent Blocked",
"detail": {
"version": 1,
"blocked_ip": "204.124.80.36",
"cluster_token": {
"uuid": "c1164b28-bace-436-ac44-4133e1d18ca5",
"description": "Default agent token",
"allowed_ip_addresses": "202.144.160.0/24",
},
"agent": {
"uuid": "0188f51c-7bc8-4b14-a702-002c485ae2dc",
"graphql_id": "QWdlbnQtLSOMTg4ZjUxYy03YmM4LTRiMTQtYTcwMi@ MDJjNDg1YWUyZGM=",
"connection_state": "disconnected",
"name": "rogue-agent-1",
"version": "3.40.0",
"token": null,
"ip_address": "127.0.0.1",
"hostname": "rogue-agent",
"pid": "26089",
"priority": 0,
"meta_data": ["queues=default"],
"connected_at": "2023-06-26 00:31:04 UTC",
"disconnected_at": "2023-06-26 00:31:18 UTC",
"Lost_at": null,
},
"organization": {
"uuid": "a98961b7-adc1-41aa-8726-cfb2c46e42e0",
"graphql_id": "T3JnYW5pemF0aW9uLS0tYTk4OTYxYjctYWRjMS00MWFhLTg3MjYtY2ZiMmM0NmU0MmUw",
"slug": "my-org"
}
}
}
Cluster Token Registration Blocked
{
"detail-type": "Cluster Token Registration Blocked",
"detail": {
"version": 1,
"blocked_ip": "204.124.80.36",
"cluster_token": {
"uuid": "c1164b28-bace-436-ac44-4133e1d18ca5",
"description": "Default agent token",
"allowed_ip_addresses": "202.144.160.0/24",
},
"organization": {
"uuid": "a98961b7-adc1-41aa-8726-cfb2c46e42e0",
"graphql_id": "T3JnYW5pemF0aW9uLS0tYTk4OTYxYjctYWRjMS00MWFhLTg3MjYtY2ZiMmM0NmU0MmUw",
"slug": "my-org"
}
}
}
Audit Event Logged
Audit log is only available to Buildkite customers on the Enterprise plan.
{
"version": "0",
"id": "8212ed90-edcc-0936-187c-d466e46575b6",
"detail-type": "Audit Event Logged",
"source": "aws.partner/buildkite.com/buildkite/0106-187c-12cd4fe",
"account": "354123020283",
"time": "2023-03-07T23:14:43Z",
"region": "us-east-1",
"resources": [],
"detail": {
"version": 1,
"organization": {
"uuid": "ae85860c-94f9-4275-91cb-3986590f45b5",
"graphql_id": "T3JnYWMDE4NjDAtNzk1YS00YWMwLWE112jUtM12jEGMzYTNkZDQx",
"slug": "buildkite"
},
"event": {
"uuid": "da55860c-94f9-4275-91cb-3986590f45b5",
"occurred_at": "2023-03-25 23:14:43 UTC",
"type": "ORGANIZATION_UPDATED",
"data": {
"name": "Buildkite"
},
"subject_type": "Organization",
"subject_uuid": "af7e863c-94f9-4275-91sb-3986590f45b5",
"subject_name": "Buildkite",
"context": "{\"request_id\":\"pemF0aW9uLStMDE4NjDAtNzk1YS00YW\",\"request_ip\":\"127.0.0.0\",\"session_key\":\"pemF0aW9uLStMDE4NjDAtNzk1YS00YW\",\"session_user_uuid\":\"da55860c-94f9-4275-91cb-3986590f45b5\",\"request_user_agent\":\"Mozilla/5.0 (Macintosh; Intel Mac OS X 10_15_7) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/110.0.0.0 Safari/537.36\",\"session_created_at\":\"2023-03-25T23:30:54.559Z\"}"
},
"actor": {
"name": "Buildkite member",
"type": null,
"uuid": "df75860c-94f9-4275-91cb-3986590f45b5"
}
}
}